The semantically correct way to remove a property from an object is to use the delete
keyword.
从对象上删除属性的语义正确方法是使用delete
关键字。
Given the object
给定对象
const car = {
color: 'blue',
brand: 'Ford'
}
you can delete a property from this object using
您可以使用以下命令从此对象中删除属性
delete car.brand
It works also expressed as:
它的工作方式还表示为:
delete car['brand']
delete car.brand
delete newCar['brand']
将属性设置为undefined (Setting a property to undefined)
If you need to perform this operation in a very optimized way, for example when you’re operating on a large number of objects in loops, another option is to set the property to undefined
.
如果您需要以非常优化的方式执行此操作,例如在循环中对大量对象进行操作时,另一种选择是将属性设置为undefined
。
Due to its nature, the performance of delete
is a lot slower than a simple reassignment to undefined
, more than 50x times slower.
由于其性质, delete
的性能要比简单地重新分配给undefined
慢很多 ,比慢得多50倍。
However, keep in mind that the property is not deleted from the object. Its value is wiped, but it’s still there if you iterate the object:
但是,请记住,该属性不会从对象中删除。 它的值被擦除了,但是如果您迭代该对象,它仍然存在:
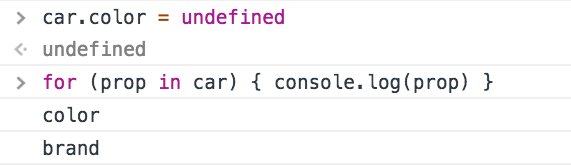
Using delete
is still very fast, you should only look into this kind of performance issues if you have a very good reason to do so, otherwise it’s always preferred to have a more clear semantic and functionality.
使用delete
仍然非常快,只有在您有充分理由这样做时,才应研究此类性能问题,否则始终首选具有更清晰的语义和功能。
删除属性而不更改对象 (Remove a property without mutating the object)
If mutability is a concern, you can create a completely new object by copying all the properties from the old, except the one you want to remove:
如果需要考虑可变性,则可以通过复制旧属性中的所有属性来创建一个全新的对象,但要删除的属性除外:
const car = {
color: 'blue',
brand: 'Ford'
}
const prop = 'color'
const newCar = Object.keys(car).reduce((object, key) => {
if (key !== prop) {
object[key] = car[key]
}
return object
}, {})
(see Object.keys()
)
(请参阅Object.keys()
)
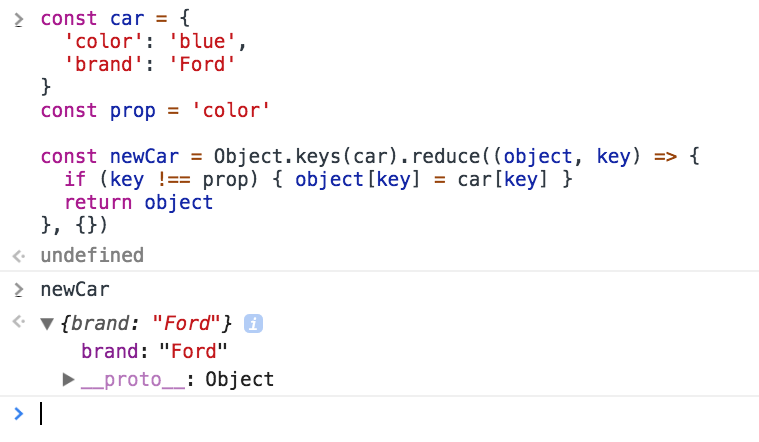
翻译自: https://flaviocopes.com/how-to-remove-object-property-javascript/