效果视频
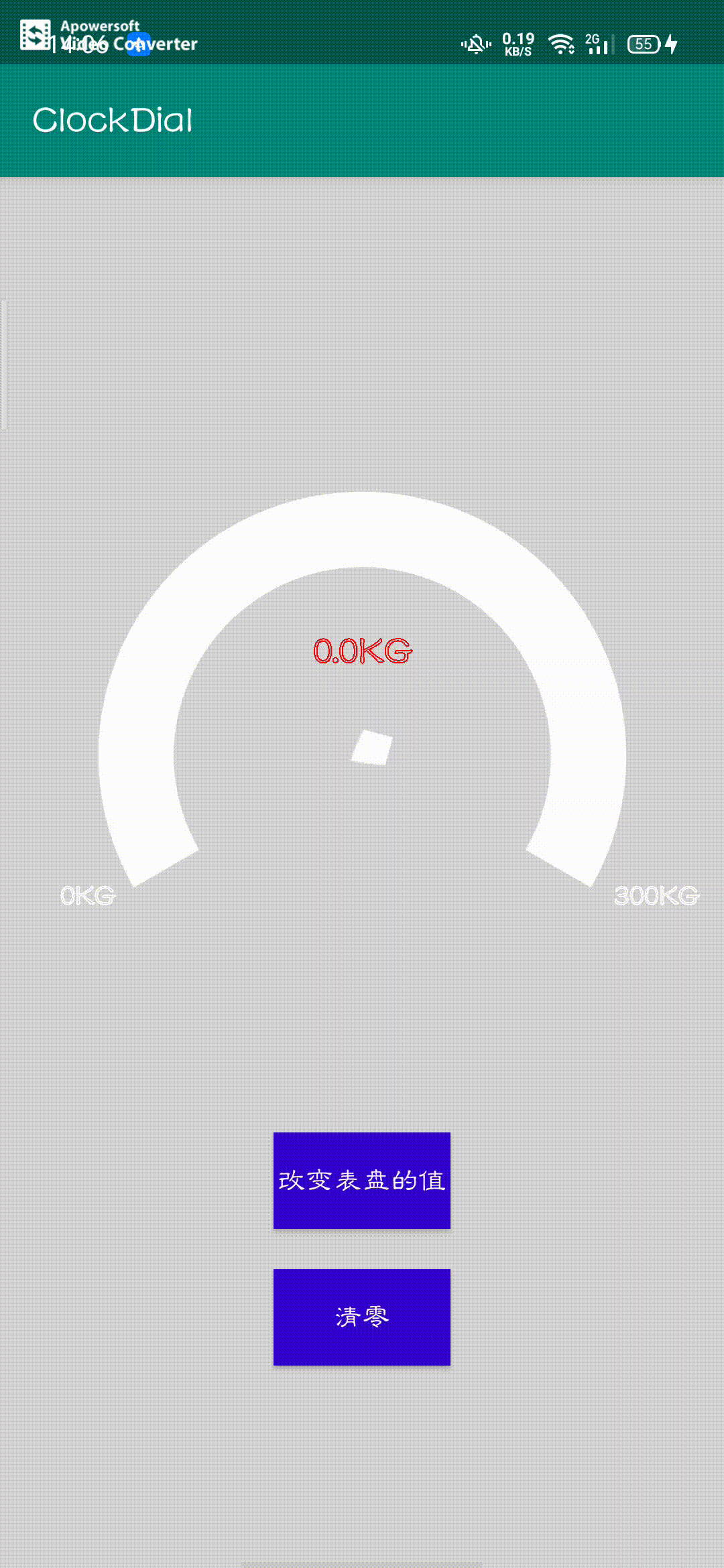
分析
起始角度
如下图所示,起点角度为150,终点角度为240
圆弧
白色圆弧为整个圆弧范围,蓝色圆弧为根据数据变动而覆盖白色圆弧,蓝色圆弧比白色圆弧大一点,突出显示
InnerArcPaint.setStrokeWidth( Width * (float)0.1 );
OuterArcPaint.setStrokeWidth( Width * (float)0.12 );
指针
中间的水滴指针是一个白色的水滴图片,下图蓝色为选择文件的背景颜色(截图),由于水滴指向-135度,将图像旋转-75度,水滴尖刚好指向150度的起点。
代码
初始化属性
private void InitPaint(){
InnerArcPaint = new Paint( );
InnerArcPaint.setColor( Color.WHITE );
InnerArcPaint.setAntiAlias( true );
InnerArcPaint.setStyle( Paint.Style.STROKE );
OuterArcPaint = new Paint( );
OuterArcPaint.setColor( Color.BLUE );
OuterArcPaint.setAntiAlias( true );
OuterArcPaint.setStyle( Paint.Style.STROKE );
OuterArcPaint.setShadowLayer( (float)10,(float)10,(float)10,Color.parseColor( "#99000000" ) );
TextPaint = new Paint( );
TextPaint.setColor( Color.RED );
TextPaint.setStyle( Paint.Style.STROKE );
TextPaint.setTextSize( 60 );
TextPaint.setStrokeWidth( 2 );
ScalePaint = new Paint( );
ScalePaint.setColor( Color.WHITE );
ScalePaint.setTextSize( 25 );
//硬件加速
setLayerType( LAYER_TYPE_SOFTWARE,null );
}
画布
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
super.onMeasure( widthMeasureSpec, heightMeasureSpec );
int Width = MeasureSpec.getSize( widthMeasureSpec );
InnerArcPaint.setStrokeWidth( Width * (float)0.1 );
OuterArcPaint.setStrokeWidth( Width * (float)0.12 );
oval = new RectF( );
oval.left = Width * (float)0.2;
oval.top = Width * (float)0.2;
oval.right = Width * (float)0.8;
oval.bottom = Width * (float)0.8;
//宽、高一致,使画布无论边长如何变化,都成为一个正方形
setMeasuredDimension( Width,Width );
}
绘制内圆弧
//绘制内圆弧
private void DrawInnerArc(Canvas canvas){
//保存之前的画布
canvas.save();
canvas.drawArc( oval, StartAngle,SweepAngle,false,InnerArcPaint);
}
绘制外圆弧
//绘制外圆弧
private void DrawOuterArc(Canvas canvas){
canvas.save();
canvas.drawArc( oval, StartAngle,SweepAngle * CurrentData / 300,false,OuterArcPaint);
}
绘制中间指针
//绘制中间指针
private void DrawArrow(Canvas canvas){
canvas.save();
Bitmap bitmap = BitmapFactory.decodeResource( getResources(),R.mipmap.waterdrop );
int width = 75;
int height = 75;
int NewWidth = (int)(getWidth() * 0.08);
float ScaleWidth = (float) (NewWidth / width);
float ScaleHeight = (float) (NewWidth / height);
Matrix matrix = new Matrix( );
//顺序不能颠倒
matrix.setRotate( -75 + (SweepAngle * CurrentData / 300),bitmap.getWidth()/2,bitmap.getHeight()/2 );
matrix.postScale( ScaleWidth,ScaleHeight );
Bitmap bitmap1 = Bitmap.createBitmap( bitmap,0,0,width,height,matrix,true );
canvas.drawBitmap( bitmap1,getWidth()/2 - bitmap1.getWidth()/2,getHeight()/2 - bitmap1.getHeight()/2,InnerArcPaint );
bitmap.recycle();
bitmap1.recycle();
}
绘制中间文字
private void DrawCurrentDataText(Canvas canvas){
canvas.save();
Rect rect = new Rect( );
String str = String.valueOf( CurrentData ) + "KG";
TextPaint.setColor( Color.RED );
TextPaint.getTextBounds( str,0,str.length(),rect );
canvas.drawText( str,getWidth()/2 - rect.width()/2,(int)(getHeight() * (float)0.38),TextPaint );
}
绘制左右两边文字
private void DrawScaleRightText(Canvas canvas){
canvas.save();
Rect rect = new Rect( );
String str = "300KG";
TextPaint.setTextSize( 45 );
TextPaint.getTextBounds( str,0,str.length(),rect );
TextPaint.setColor( Color.WHITE );
canvas.drawText( str,getWidth()-getWidth()/6,(getHeight()/2+getWidth()/5) ,TextPaint );
}
private void DrawScaleLeftText(Canvas canvas){
canvas.save();
Rect rect = new Rect( );
String str = "0KG";
TextPaint.setTextSize( 45 );
TextPaint.getTextBounds( str,0,str.length(),rect );
TextPaint.setColor( Color.WHITE );
canvas.drawText( str,(getWidth()/2-(getWidth()/3 + 75)),(getHeight()/2+getWidth()/5) ,TextPaint );
}
动画
public void SetCurrentData(final float data, TimeInterpolator interpolator){
long time = ( (long)Math.abs( data- CurrentData ) *20);
final ValueAnimator valueAnimator = ValueAnimator.ofFloat( CurrentData,data ).setDuration( time );
valueAnimator.setInterpolator( interpolator );
valueAnimator.addUpdateListener( new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animation) {
CustomView_ClockDial.this.CurrentData = (float)valueAnimator.getAnimatedValue();
invalidate();
}
} );
valueAnimator.start();
}
全部代码
public class CustomView_ClockDial extends View {
//内圆弧画笔
private Paint InnerArcPaint;
//外圆弧画笔
private Paint OuterArcPaint;
//文字画笔
private Paint TextPaint;
//刻度画笔
private Paint ScalePaint;
//圆弧范围
private RectF oval;
//当前数据
private float CurrentData = 0;
//起点角度
private float StartAngle = 150;
//终点角度
private float SweepAngle = 240;
public CustomView_ClockDial(Context context) {
super( context );
InitPaint();
}
public CustomView_ClockDial(Context context, @Nullable AttributeSet attrs) {
super( context, attrs );
InitPaint();
}
public CustomView_ClockDial(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super( context, attrs, defStyleAttr );
InitPaint();
}
private void InitPaint(){
InnerArcPaint = new Paint( );
InnerArcPaint.setColor( Color.WHITE );
InnerArcPaint.setAntiAlias( true );
InnerArcPaint.setStyle( Paint.Style.STROKE );
OuterArcPaint = new Paint( );
OuterArcPaint.setColor( Color.BLUE );
OuterArcPaint.setAntiAlias( true );
OuterArcPaint.setStyle( Paint.Style.STROKE );
OuterArcPaint.setShadowLayer( (float)10,(float)10,(float)10,Color.parseColor( "#99000000" ) );
TextPaint = new Paint( );
TextPaint.setColor( Color.RED );
TextPaint.setStyle( Paint.Style.STROKE );
TextPaint.setTextSize( 60 );
TextPaint.setStrokeWidth( 2 );
ScalePaint = new Paint( );
ScalePaint.setColor( Color.WHITE );
ScalePaint.setTextSize( 25 );
//硬件加速
setLayerType( LAYER_TYPE_SOFTWARE,null );
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
super.onMeasure( widthMeasureSpec, heightMeasureSpec );
int Width = MeasureSpec.getSize( widthMeasureSpec );
InnerArcPaint.setStrokeWidth( Width * (float)0.1 );
OuterArcPaint.setStrokeWidth( Width * (float)0.12 );
oval = new RectF( );
oval.left = Width * (float)0.2;
oval.top = Width * (float)0.2;
oval.right = Width * (float)0.8;
oval.bottom = Width * (float)0.8;
//宽、高一致,使画布无论边长如何变化,都成为一个正方形
setMeasuredDimension( Width,Width );
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw( canvas );
DrawInnerArc(canvas);
DrawOuterArc(canvas);
DrawArrow(canvas);
DrawCurrentDataText(canvas);
DrawScaleRightText(canvas);
DrawScaleLeftText(canvas);
}
//绘制内圆弧
private void DrawInnerArc(Canvas canvas){
//保存之前的画布
canvas.save();
canvas.drawArc( oval, StartAngle,SweepAngle,false,InnerArcPaint);
}
//绘制外圆弧
private void DrawOuterArc(Canvas canvas){
canvas.save();
canvas.drawArc( oval, StartAngle,SweepAngle * CurrentData / 300,false,OuterArcPaint);
}
//绘制中间指针
private void DrawArrow(Canvas canvas){
canvas.save();
Bitmap bitmap = BitmapFactory.decodeResource( getResources(),R.mipmap.waterdrop );
int width = 75;
int height = 75;
int NewWidth = (int)(getWidth() * 0.08);
float ScaleWidth = (float) (NewWidth / width);
float ScaleHeight = (float) (NewWidth / height);
Matrix matrix = new Matrix( );
//顺序不能颠倒
matrix.setRotate( -75 + (SweepAngle * CurrentData / 300),bitmap.getWidth()/2,bitmap.getHeight()/2 );
matrix.postScale( ScaleWidth,ScaleHeight );
Bitmap bitmap1 = Bitmap.createBitmap( bitmap,0,0,width,height,matrix,true );
canvas.drawBitmap( bitmap1,getWidth()/2 - bitmap1.getWidth()/2,getHeight()/2 - bitmap1.getHeight()/2,InnerArcPaint );
bitmap.recycle();
bitmap1.recycle();
}
private void DrawCurrentDataText(Canvas canvas){
canvas.save();
Rect rect = new Rect( );
String str = String.valueOf( CurrentData ) + "KG";
TextPaint.setColor( Color.RED );
TextPaint.getTextBounds( str,0,str.length(),rect );
canvas.drawText( str,getWidth()/2 - rect.width()/2,(int)(getHeight() * (float)0.38),TextPaint );
}
private void DrawScaleRightText(Canvas canvas){
canvas.save();
Rect rect = new Rect( );
String str = "300KG";
TextPaint.setTextSize( 45 );
TextPaint.getTextBounds( str,0,str.length(),rect );
TextPaint.setColor( Color.WHITE );
canvas.drawText( str,getWidth()-getWidth()/6,(getHeight()/2+getWidth()/5) ,TextPaint );
}
private void DrawScaleLeftText(Canvas canvas){
canvas.save();
Rect rect = new Rect( );
String str = "0KG";
TextPaint.setTextSize( 45 );
TextPaint.getTextBounds( str,0,str.length(),rect );
TextPaint.setColor( Color.WHITE );
canvas.drawText( str,(getWidth()/2-(getWidth()/3 + 75)),(getHeight()/2+getWidth()/5) ,TextPaint );
}
public void SetCurrentData(final float data, TimeInterpolator interpolator){
long time = ( (long)Math.abs( data- CurrentData ) *20);
final ValueAnimator valueAnimator = ValueAnimator.ofFloat( CurrentData,data ).setDuration( time );
valueAnimator.setInterpolator( interpolator );
valueAnimator.addUpdateListener( new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animation) {
CustomView_ClockDial.this.CurrentData = (float)valueAnimator.getAnimatedValue();
invalidate();
}
} );
valueAnimator.start();
}
}