python列表连接
Python join list means concatenating a list of strings with a specified delimiter to form a string. Sometimes it’s useful when you have to convert list to string. For example, convert a list of alphabets to a comma-separated string to save in a file.
Python连接列表意味着将字符串列表与指定的分隔符连接起来以形成字符串。 有时在必须将列表转换为字符串时很有用。 例如,将字母列表转换为逗号分隔的字符串以保存在文件中。
Python连接列表 (Python Join List)
We can use python string join() function to join a list of strings. This function takes iterable
as argument and List is an interable, so we can use it with List.
我们可以使用python string join()函数来连接字符串列表。 该函数将iterable
作为参数,并且List是一个可互操作的,因此我们可以将其与List一起使用。
Also, the list should contain strings, if you will try to join a list of ints then you will get an error message as TypeError: sequence item 0: expected str instance, int found
.
另外,该列表应包含字符串,如果您尝试加入一个ints列表,则将收到错误消息,类型错误TypeError: sequence item 0: expected str instance, int found
。
Let’s look at a short example for joining list in python to create a string.
让我们看一个简短的示例,在python中加入list来创建一个字符串。
vowels = ["a", "e", "i", "o", "u"]
vowelsCSV = ",".join(vowels)
print("Vowels are = ", vowelsCSV)
When we run the above program, it will produce the following output.
当我们运行上面的程序时,它将产生以下输出。
Vowels are = a,e,i,o,u
Python连接两个字符串 (Python join two strings)
We can use join() function to join two strings too.
我们也可以使用join()函数来连接两个字符串。
message = "Hello ".join("World")
print(message) #prints 'Hello World'
为什么join()函数在String中而不在List中? (Why join() function is in String and not in List?)
One question arises with many python developers is why the join() function is part of String and not list. Wouldn’t below syntax be more easy to remember and use?
许多python开发人员都会想到一个问题,为什么join()函数是String的一部分而不是list的一部分。 下面的语法难道不容易记住和使用吗?
vowelsCSV = vowels.join(",")
There is a popular StackOverflow question around this, here I am listing the most important points from the discussions that makes total sense to me.
围绕此有一个流行的StackOverflow问题 ,在这里,我列出了讨论中最有意义的要点。
多种数据类型的联接列表 (Joining list of multiple data-types)
Let’s look at a program where we will try to join list items having multiple data types.
让我们看一个程序,在该程序中,我们将尝试加入具有多种数据类型的列表项。
names = ['Java', 'Python', 1]
delimiter = ','
single_str = delimiter.join(names)
print('String: {0}'.format(single_str))
Let’s see the output for this program:
让我们看一下该程序的输出:
This was just a demonstration that a list which contains multiple data-types cannot be combined into a single String with join()
function. List must contain only the String values.
这只是一个演示,表明无法使用join()
函数将包含多个数据类型的列表组合为单个String 。 列表必须仅包含String值 。
使用连接函数分割字符串 (Split String using join function)
We can use join()
function to split a string with specified delimiter too.
我们也可以使用join()
函数来使用指定的分隔符分割字符串。
names = 'Python'
delimiter = ','
single_str = delimiter.join(names)
print('String: {0}'.format(single_str))
This shows that when String is passed as an argument to join() function, it splits it by character and with the specified delimiter.
这表明,当将String作为参数传递给join()函数时,它将按字符并使用指定的分隔符对其进行拆分。
使用split()函数 (Using split() function)
Apart from splitting with the join()
function, split()
function can be used to split a String as well which works almost the same way as the join()
function. Let’s look at a code snippet:
除了使用join()
函数进行split()
, split()
函数还可用于拆分String,其工作方式与join()
函数几乎相同。 让我们看一下代码片段:
names = ['Java', 'Python', 'Go']
delimiter = ','
single_str = delimiter.join(names)
print('String: {0}'.format(single_str))
split = single_str.split(delimiter)
print('List: {0}'.format(split))
Let’s see the output for this program:
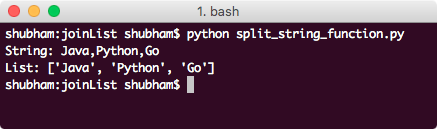
We used the same delimiter to split the String again to back to the original list.
让我们看一下该程序的输出:
我们使用相同的定界符再次将String拆分为原始列表。
仅拆分n次 (Splitting only n times)
The split()
function we demonstrated in the last example also takes an optional second argument which signifies the number of times the splot operation should be performed. Here is a sample program to demonstrate its usage:
我们在上一个示例中演示的split()
函数还采用了可选的第二个参数,该参数表示应执行splot操作的次数。 这是一个示例程序来演示其用法:
names = ['Java', 'Python', 'Go']
delimiter = ','
single_str = delimiter.join(names)
print('String: {0}'.format(single_str))
split = single_str.split(delimiter, 1)
print('List: {0}'.format(split))
Let’s see the output for this program:
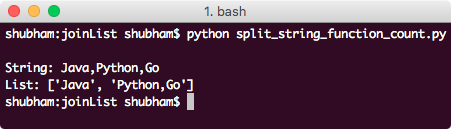
This time, split operation was performed only one time as we provided in the
split()
function parameter.
让我们看一下该程序的输出:
这次,我们仅在split()
函数参数中提供了一次拆分操作。
That’s all for joining a list to create a string in python and using split() function to get the original list again.
这就是为了加入列表以在python中创建字符串并使用split()函数再次获取原始列表而已。
python列表连接