pom.xml文件添加依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
在请求实体类在校验绑定
import io.swagger.annotations.ApiModelProperty;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
@ApiModel(value = "参数校验请求类")
public class TestVaild {
@NotNull(message = "测试字符串不能为空")
@Size(min = 1, message = "测试字符串不能为空")
@ApiModelProperty(value = "测试字符串")
private String testString;
public String getTestString() {
return testString;
}
public void setTestString(String testString) {
this.testString = testString;
}
}
- valid注解类型,找到其中一个注解的位置就可以看到其他注解
使用 @Valid 注解,校验bean传递参数
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import org.springframework.http.MediaType;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.validation.Valid;
@RestController
@RequestMapping(value = "valid", produces = MediaType.APPLICATION_PROBLEM_JSON_VALUE)
@Api(tags = "测试valid")
public class TestValidController {
@PostMapping("testVaild")
@ApiOperation(value = "获取用户信息")
public String getUserInfo(@RequestBody @Valid TestVaild req) {
return "hello word";
}
}
接口调用测试验证效果
- 返回404
- 看一下后端输出实际错误
WARN o.s.w.s.m.s.DefaultHandlerExceptionResolver - Resolved [org.springframework.web.bind.MethodArgumentNotValidException: Validation failed for argument [0] in public java.lang.String com.kangqiao.openapi.controller.TestValidController.getUserInfo(com.kangqiao.openapi.dto.req.TestValidReq): [Field error in object 'testValidReq' on field 'testString': rejected value []; codes [Size.testValidReq.testString,Size.testString,Size.java.lang.String,Size]; arguments [org.springframework.context.support.DefaultMessageSourceResolvable: codes [testValidReq.testString,testString]; arguments []; default message [testString],2147483647,1]; default message [测试字符串不能为空]] ]
- 其实后端是校验参数未通过的,我们对异常进行捕获处理
全局异常处理
- 对MethodArgumentNotValidException异常进行处理
@RestControllerAdvice
public class ExceptionConfig {
private Logger log = LoggerFactory.getLogger(this.getClass());
@ExceptionHandler(MethodArgumentNotValidException.class)
@ResponseBody
public BaseResp requestParameterException(MethodArgumentNotValidException e) {
log.error(e.getMessage());
return new BaseResp(false, "fail", 500, e.getBindingResult().getFieldError().getDefaultMessage());
}
}
异常捕获之后,检验结果
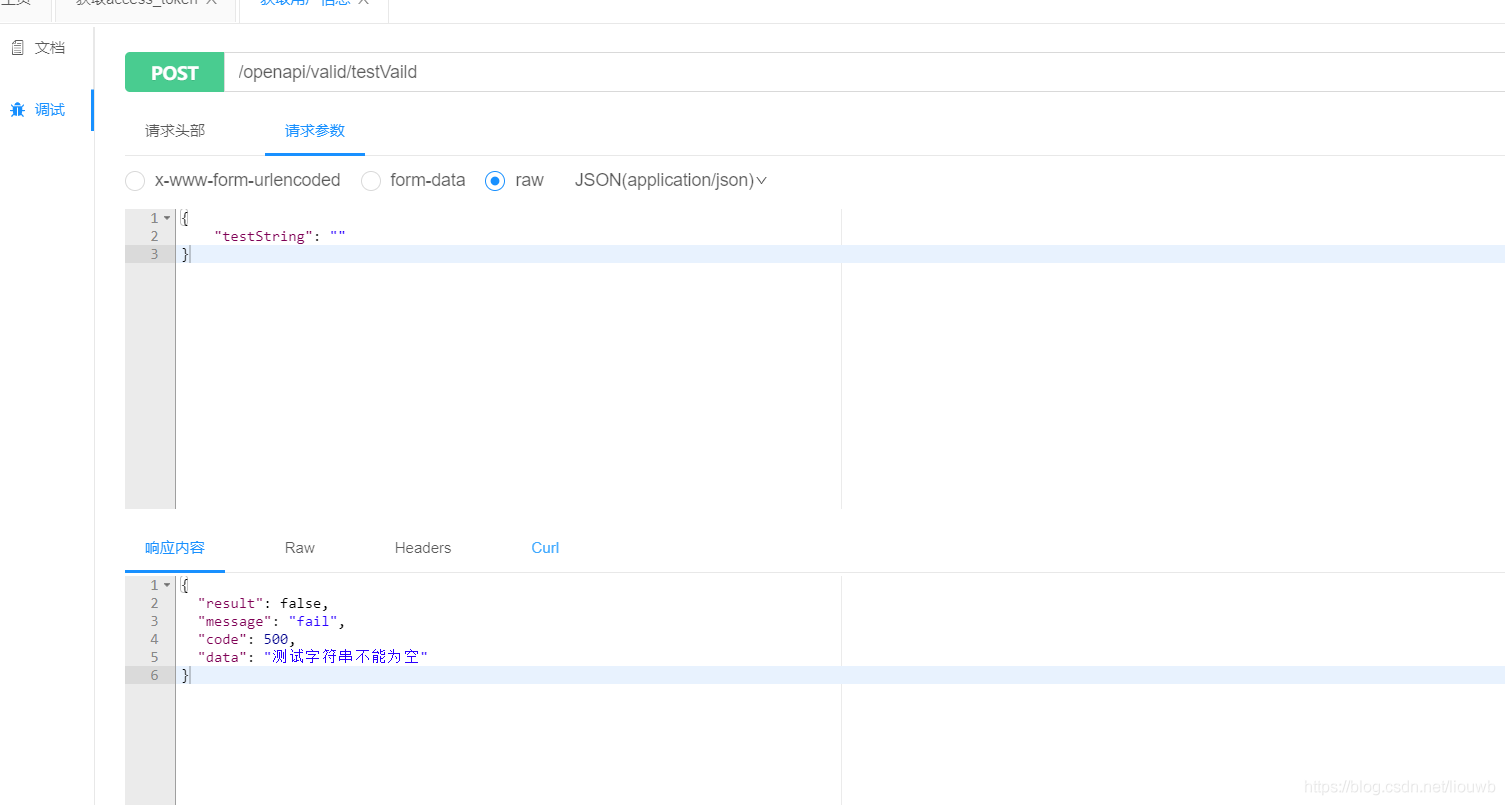
validtor国际化配置
默认配置
- @Valid 的 message 国际化资源文件默认放在
resources/ValidationMessages.properties
中
- 国际化配置使用
自定义配置文件位置
- 自定义配置文件需要重新WebMvcConfigurationSupport的==getValidator()==方法
方式一、在java代码中指定valid文件
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.support.ResourceBundleMessageSource;
import org.springframework.validation.Validator;
import org.springframework.validation.beanvalidation.LocalValidatorFactoryBean;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurationSupport;
@Configuration
public class MessageConfig extends WebMvcConfigurationSupport {
@Bean
public ResourceBundleMessageSource getMessageSource() {
ResourceBundleMessageSource rbms = new ResourceBundleMessageSource();
rbms.setDefaultEncoding("UTF-8");
rbms.setBasenames("i18n/ValidationMessages_zh_CN");
return rbms;
}
@Override
public Validator getValidator() {
LocalValidatorFactoryBean validator = new LocalValidatorFactoryBean();
validator.setValidationMessageSource(getMessageSource());
return validator;
}
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/**").addResourceLocations(
"classpath:/static/");
registry.addResourceHandler("doc.html").addResourceLocations(
"classpath:/META-INF/resources/");
registry.addResourceHandler("/webjars/**").addResourceLocations(
"classpath:/META-INF/resources/webjars/");
super.addResourceHandlers(registry);
}
}
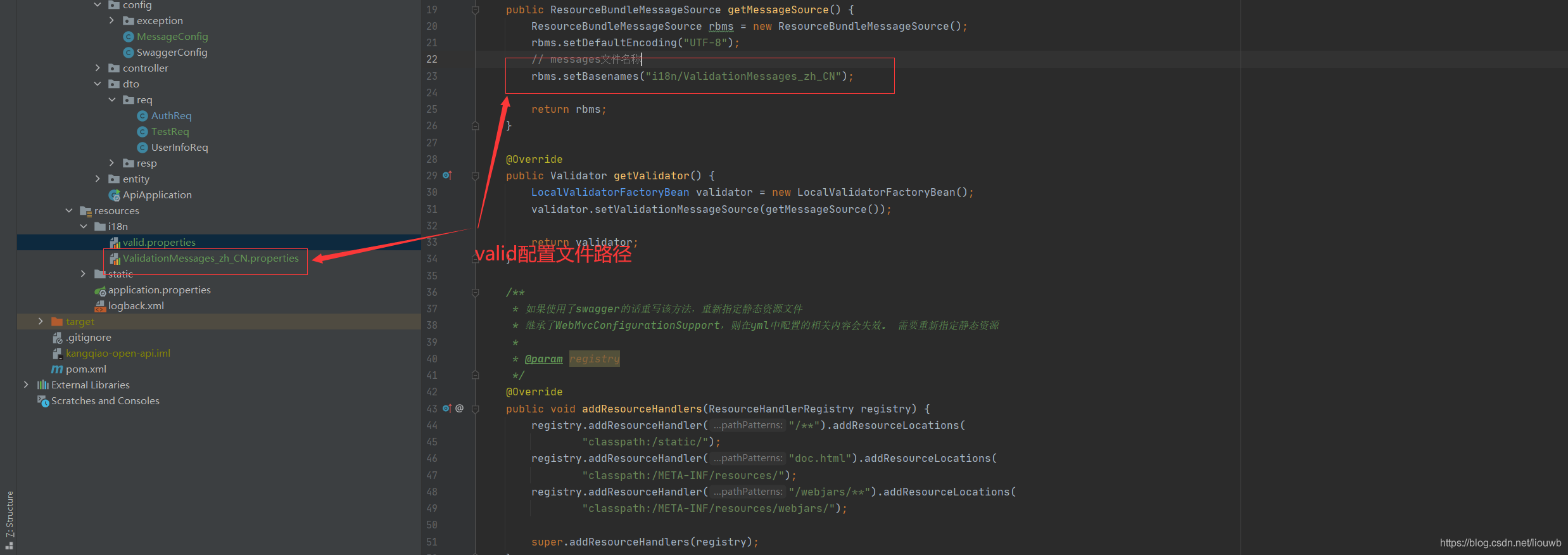
方式二、在application.properties或者application.yml文件指定valid文件位置
spring.messages.encoding=UTF-8
spring.messages.basename=i18n/ValidationMessages_zh_CN
-
WebMvcConfigurationSupport的getValidator() 方法改动如下
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.MessageSource;
import org.springframework.context.annotation.Configuration;
import org.springframework.validation.Validator;
import org.springframework.validation.beanvalidation.LocalValidatorFactoryBean;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurationSupport;
@Configuration
public class MessageConfig extends WebMvcConfigurationSupport {
@Autowired
private MessageSource messageSource;
@Override
public Validator getValidator() {
LocalValidatorFactoryBean validator = new LocalValidatorFactoryBean();
validator.setValidationMessageSource(messageSource);
return validator;
}
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/**").addResourceLocations(
"classpath:/static/");
registry.addResourceHandler("doc.html").addResourceLocations(
"classpath:/META-INF/resources/");
registry.addResourceHandler("/webjars/**").addResourceLocations(
"classpath:/META-INF/resources/webjars/");
super.addResourceHandlers(registry);
}
}
验证valid国际化自定义文件,测试结果,出现乱码
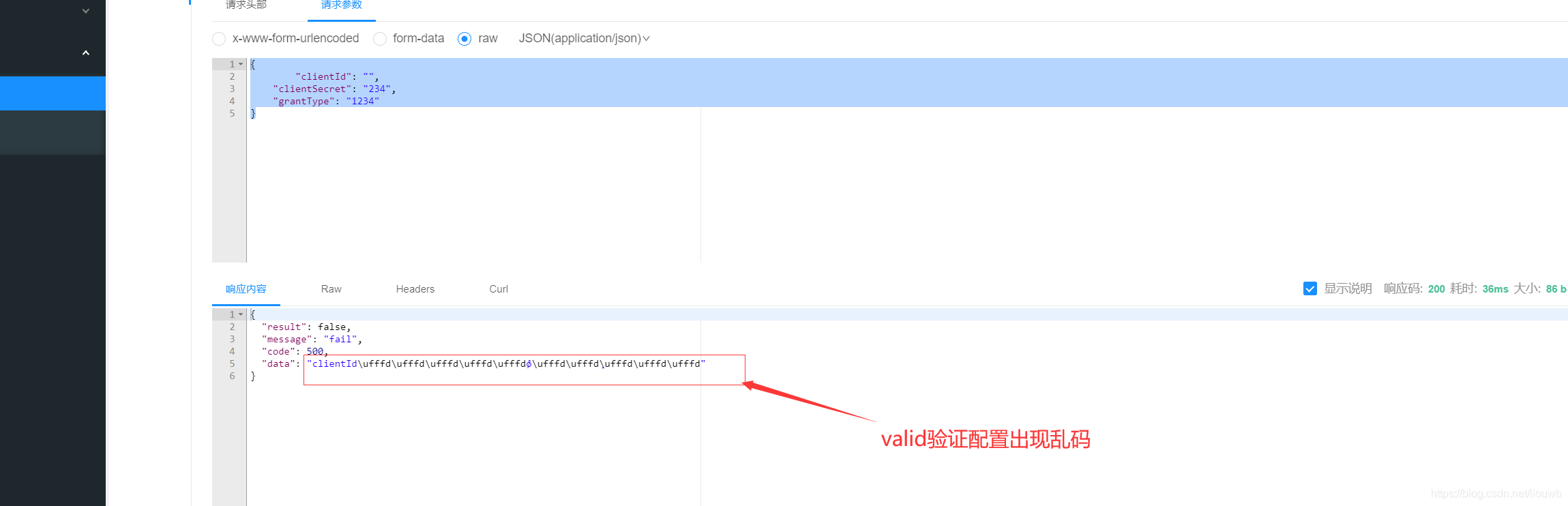
- 乱码原因是由于idea编辑器导致的,修改idea editer的配置
- 编码修改之后,正常显示