目录
编辑编辑编辑3.在@RequestMapping注解的基础上,结合请求方式的派生注解 编辑
五、@RequestMapping注解的params属性(了解)
六、@RequestMapping注解的headers属性(了解)
@RequestMapping的源码
//
// Source code recreated from a .class file by IntelliJ IDEA
// (powered by FernFlower decompiler)
//
package org.springframework.web.bind.annotation;
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import org.springframework.core.annotation.AliasFor;
@Target({ElementType.TYPE, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Mapping
public @interface RequestMapping {
String name() default "";
@AliasFor("path") //value属性,别名:path,通过请求的请求路径匹配
String[] value() default {};
@AliasFor("value")
String[] path() default {};
RequestMethod[] method() default {}; //通过请求方式匹配
String[] params() default {}; //通过请求参数匹配
String[] headers() default {}; //通过请求头信息匹配
String[] consumes() default {};
String[] produces() default {};
}
一、@RequestMapping注解的功能
将请求和处理请求的控制器方法关联起来,建立映射关系。
SpringMVC 接收到指定的请求,就会来找到在映射关系中对应的控制器方法来处理这个请求。
二、@RequestMapping注解的位置
- 标识类:设置映射请求的请求路径的初始信息
- 标识方法:设置映射请求请求路径的具体信息
package com.atguigu.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/test")
public class TestRequestMappingController {
//此时控制器方法所匹配的请求路径为/test/hello
@RequestMapping("/hello")
public String hello(){
return "success";
}
}
三、@RequestMapping注解的value属性
value属性通过请求的请求地址匹配请求映射
@RequestMapping注解的value属性是一个字符串类型的数组,表示该请求映射能够匹配多个请求地址所对应的请求,即当前浏览器所发送请求的请求路径匹配value属性中的任何一个值,则当前请求就会被注解所标识的方法进行处理
@RequestMapping注解的value属性必须设置,至少通过请求地址匹配请求映射
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
//@RequestMapping("/test")
public class TestRequestMappingController {
//此时控制器方法所匹配的请求路径为/test/hello
@RequestMapping({"/hello","/abc"})
public String hello(){
return "success";
}
}
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a>
<a th:href="@{/hello}">测试@RequestMapping注解的value属性</a>
</body>
</html>
四、@RequestMapping注解的method属性
method属性通过请求的请求方式(get或post)匹配请求映射
@RequestMapping注解的method属性是一个RequestMethod类型的数组,表示该请求映射能够匹配多种请求方式的请求,则当前请求就会被注解所标识的方法进行处理
若浏览器所发送的请求的请求路径和@RequestMapping注解的value属性匹配,但是请求方式不满足method属性,则浏览器报错 405:Request method 'XXXX' not supported
⚪RequestMethod 的源码
package org.springframework.web.bind.annotation;
public enum RequestMethod {
GET,
HEAD,
POST,
PUT,
PATCH,
DELETE,
OPTIONS,
TRACE;
private RequestMethod() {
}
}
示例:
1.GET方法
@Controller
//@RequestMapping("/test")
public class TestRequestMappingController {
//此时控制器方法所匹配的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
method = RequestMethod.GET
)
public String hello(){
return "success";
}
}
2.POST方法
@Controller
//@RequestMapping("/test")
public class TestRequestMappingController {
//此时控制器方法所匹配的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
method = RequestMethod.POST
)
public String hello(){
return "success";
}
}
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
<a th:href="@{/hello}">测试@RequestMapping注解的value属性</a><br>
<form th:action="@{/hello}">
<input type="submit" value="测试@RequestMapping注解的method属性">
</form>
</body>
</html>
此时点击,报405错误
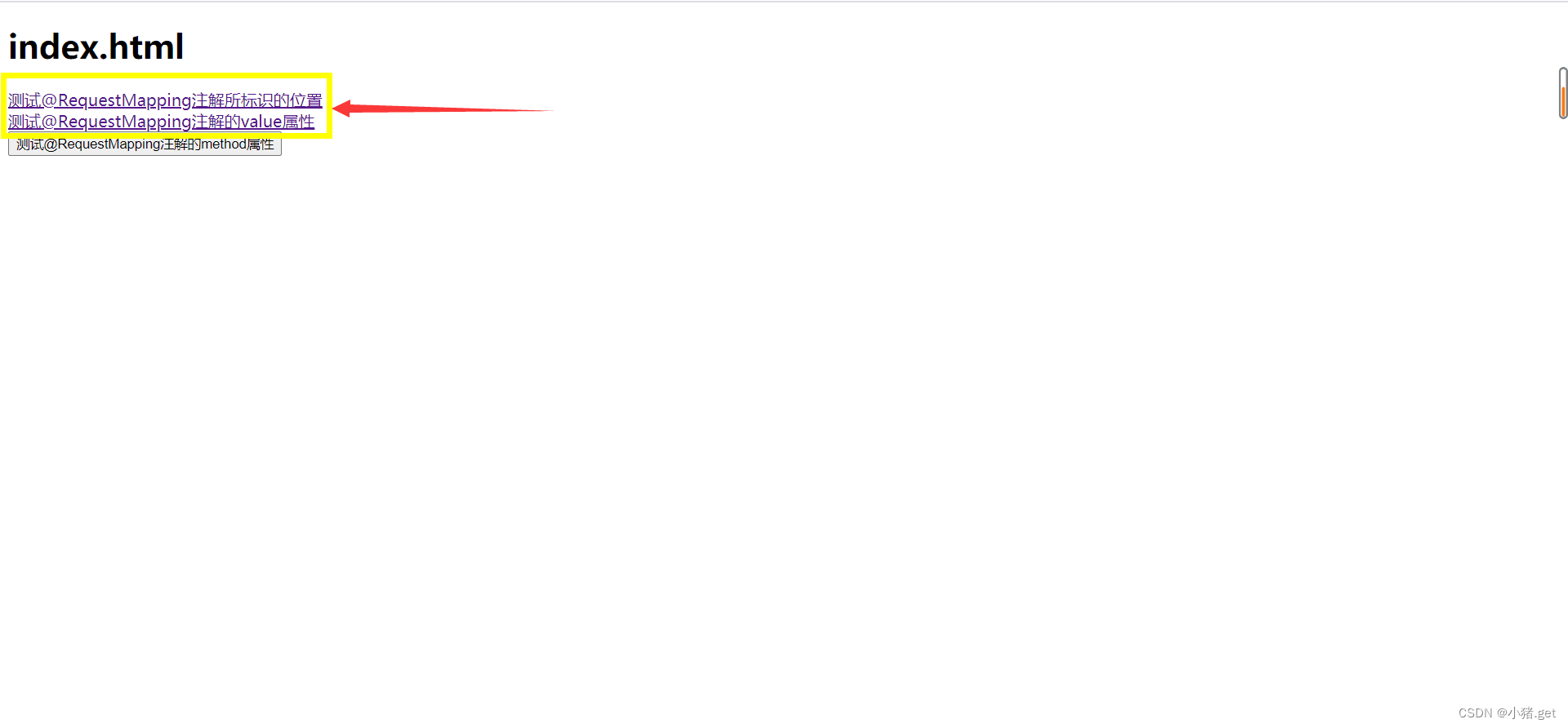
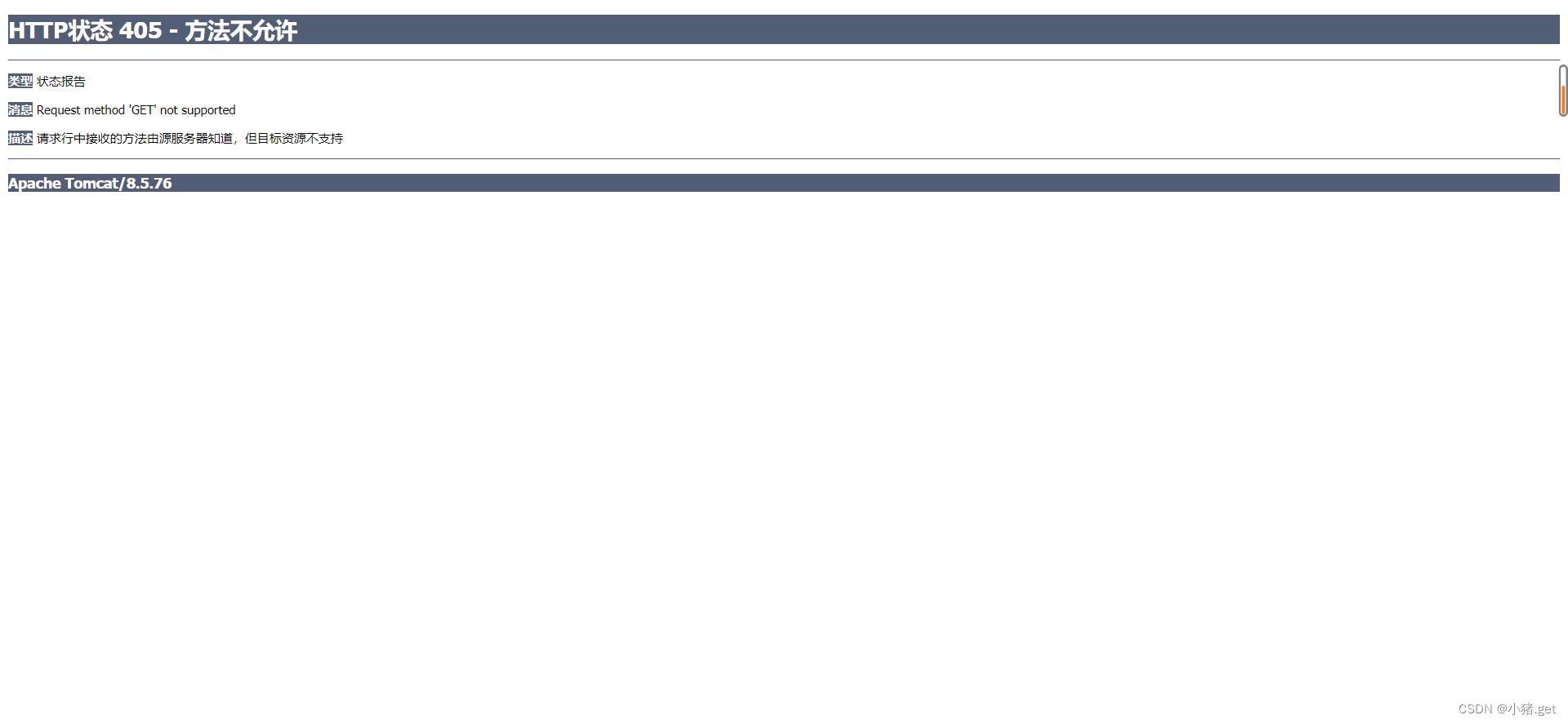
3.在@RequestMapping注解的基础上,结合请求方式的派生注解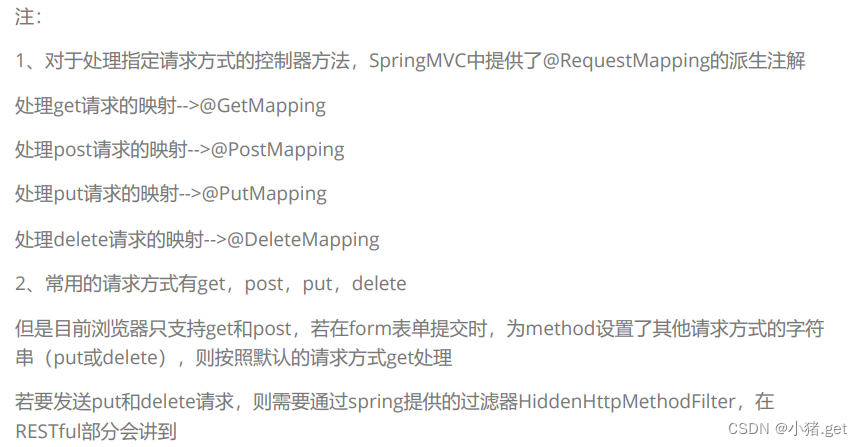
五、@RequestMapping注解的params属性(了解)
@RequestMapping注解的params属性通过请求的请求参数匹配请求映射 即浏览器发送的请求的请求参数必须满足params属性的设置,是一个字符串类型的数组
可以通过四种表达式设置请求参数和请求映射的匹配关系
- "param":表示当前所匹配请求的请求参数中必须携带param请求参数
- "!param":表示当前所匹配请求的请求参数中必须不能携带param请求参数
- "param=value":表示当前所匹配请求的请求参数中必须携带param请求参数且param=value
- "param!=value":表示当前所匹配请求的请求参数中可以不携带param,但是若携带,值一定不能时value
⭐页面报错400
@Controller
//@RequestMapping("/test")
public class TestRequestMappingController {
//此时控制器方法所匹配的请求路径为/test/hello
@RequestMapping(
value = {"/hello","/abc"},
method = {RequestMethod.POST,RequestMethod.GET},
//必须携带username,不能携带password,age=20,gender不为女
params = {"username","!password","age=20","gender!=女"}
)
public String hello(){
return "success";
}
}
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h1>index.html</h1>
<a th:href="@{/hello}">测试@RequestMapping注解所标识的位置</a><br>
<a th:href="@{/hello}">测试@RequestMapping注解的value属性</a><br>
<form th:action="@{/hello}" method="post">
<input type="submit" value="测试@RequestMapping注解的method属性">
</form>
<a th:href="@{/hello?username=admin}">测试@RequestMapping注解的params属性</a><br>
<a th:href="@{/hello(username='admin')}">测试@RequestMapping注解的params属性</a><br>
</body>
</html>
六、@RequestMapping注解的headers属性(了解)
@RequestMapping注解的headers属性通过请求的请求头信息匹配请求映射 即浏览器发送的请求的请求参数必须满足headers属性的设置,是一个字符串类型的数组
可以通过四种表达式设置请求头信息和请求映射的匹配关系
- "header":要求请求映射所匹配的请求必须携带header请求头信息
- "!header":要求请求映射所匹配的请求必须不能携带header请求头信息
- "header=value":要求请求映射所匹配的请求必须携带header请求头信息且header=value
- "header!=value":要求请求映射所匹配的请求必须携带header请求头信息且header!=value
若当前请求满足@RequestMapping注解的value和method属性,但是不满足headers属性,此时页面 显示404错误,即资源未找到
七、SpringMVC支持ant风格的路径
在@RequestMapping注解的value属性值中设置一些特殊字符
- ?:表示任意的单个字符(不包括 ?)
@RequestMapping("/a?a/test/ant")
public String testAnt(){
return "success";
}
- * :表示任意的0个或多个字符(不包括 ?和 / )
- ** :表示任意层数的任意目录
注意:在使用**时,只能使用/**/xxx的方式,**之间不允许出现其它的字符,只能将**写在双斜线中
八、SpringMVC支持路径中的占位符(重点)
原始方式:/deleteUser?id=1
rest方式:/user/delete/1
SpringMVC路径中的占位符常用于RESTful风格中
当请求路径中将某些数据通过路径的方式传输到服务器中,就可以在相应的@RequestMapping注解的value属性中通过占位符{xxx}表示传输的数据,再通过@PathVariable注解,将占位符所表示的数据赋值给控制器方法的形参
@RequestMapping("/test/rest/{id}")
public String testRest(@PathVariable("id") Integer id){
System.out.println("id:" + id);
return "success";
}
html:
<a th:href="@{/test/rest/1}">测试@RequestMapping注解的value属性中的占位符</a><br>