用户注册
//注册用户验证
public void regist()
{
string userName = Txt_userName.Text.Trim();
string passWord = Txt_passWord.Text.Trim();
try
{
if (userName != "" && passWord != "")
{
SqlConnection cn = new SqlConnection(cnstr);
cn.Open();
string sql = "insert into Table_login values('" + userName + "','" + passWord + "')";
SqlCommand cmd = new SqlCommand(sql, cn);
int count = cmd.ExecuteNonQuery();
if (count > 0)
{
MessageBox.Show("注册成功!", "提示");
resetTxt();
}
else
MessageBox.Show("注册失败", "提示");
cn.Dispose();
}
else
{
MessageBox.Show("用户名不能为空,且两次密码输入必须一致!", "提示");
}
}
catch (Exception e1)
{
MessageBox.Show(e1.Message, "提示");
}
}
//注册的数据库操作
private void Btn_register_Click(object sender, EventArgs e)
{
string userName = Txt_userName.Text.Trim();
SqlConnection cn = new SqlConnection(cnstr);
cn.Open();
string sql = "select count(*) from Table_login where userName='" + userName + "'";
SqlCommand cmd = new SqlCommand(sql, cn);
int count = (int)cmd.ExecuteScalar();
if (count > 0)
{
MessageBox.Show("注册失败,该用户已存在!", "提示");
}
else
{
regist();
}
cn.Dispose();
cn.Close();
}
//重置用户名、密码的方法
public void resetTxt()
{
this.Txt_userName.Text = "";
this.Txt_passWord.Text = "";
}
登录验证
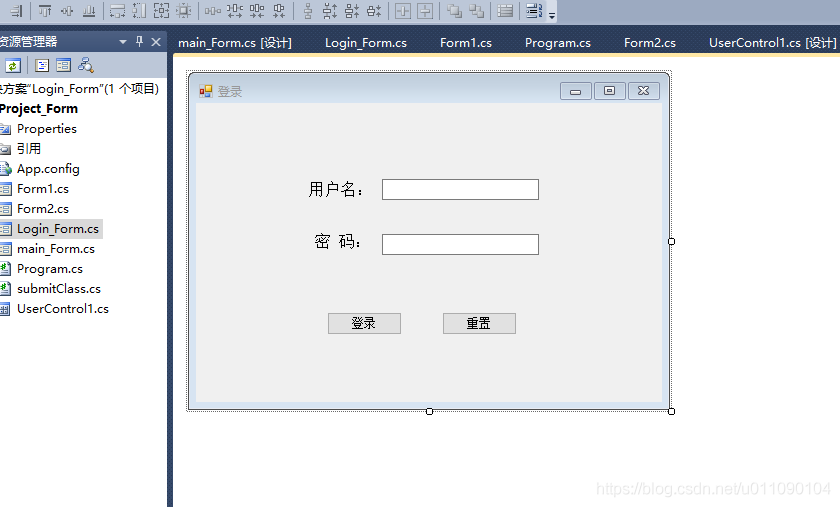
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.Sql;
using System.Data.SqlClient;
namespace Login_Form
{
public partial class Login_Form : Form
{
public Login_Form()
{
InitializeComponent();
}
public static String cnstr = "Integrated Security=SSPI;Persist Security Info=False;User ID=sa;Initial Catalog=Db_net;Data Source=.";
//登录验证
private void login_Btn_Click(object sender, EventArgs e)
{
string userName = userName_Txt.Text.Trim();
string passWord = pwd_Txt.Text.Trim();
try
{
SqlConnection cn = new SqlConnection(cnstr);
cn.Open();
string sql = "select count(*) from UserTable where userName='" + userName + "'and passWord='" + passWord + "'";
SqlCommand cmd = new SqlCommand(sql, cn);
int count = (int)cmd.ExecuteScalar();
if (count > 0)
{
MessageBox.Show("登录成功", "提示");
main_Form m1 = new main_Form();
m1.ShowDialog();
this.Hide();
}
else
{
MessageBox.Show("用户名或密码错误", "提示");
}
}
catch (Exception e1)
{
MessageBox.Show("'"+ e1 +"'", "提示");
}
}
//重置用户名、密码的方法
public void resetTxt()
{
this.userName_Txt.Text = "";
this.pwd_Txt.Text = "";
}
//重置用户名、密码
private void reset_Btn_Click(object sender, EventArgs e)
{
resetTxt();
}
}
}