介绍
模板需求说明
开发中经常遇到前端传递过来的JSON串的转换,后端需要解析成对象,有解析成List的,也有解析成Map的。
我们对Fastjson并不陌生,Fastjson 是阿里巴巴的开源JSON解析库。Fastjson可以看解析JSON格式的字符串,支持后端将Java Bean序列化成JSON字符串供给前端使用,也可以从前端传递过来的JSON字符串反序列化成Java Bean供给后端逻辑使用。
Fastjson依赖
com.alibabafastjson1.2.70
低版本漏洞
由于低版本(version <= 1.2.68
)的fastjson存在远程代码执行漏洞,该bug可以被利用直接获取服务器权限。
漏洞修复方案
引入高版本后(version >= 1.2.69
),前后端交互的bean中会出现循环引用,因此我们需要在工程的Application类中main函数中添加以下代码禁止循环引用检测。
JSON.DEFAULT_GENERATE_FEATURE |= SerializerFeature.DisableCircularReferenceDetect.getMask();
除了引入高版本,我们还可以通过配置开启SafeMode来防护攻击,完全禁用autoType,无视白名单,这会对业务有一定影响。
ParserConfig.getGlobalInstance.setSafeMode(true);
解析模板
Response获取成String
public static String getResponseAsString(final HttpResponse response) {
try {
return EntityUtils.toString(response.getEntity(), "UTF-8");
} catch (final ParseException e) {
throw new RuntimeException(e);
} catch (final IOException e) {
throw new RuntimeException(e);
}
}
JSON串解析
若已通过上述的Response方法转换成了String类型字符串为appsStr
。
JSON示例
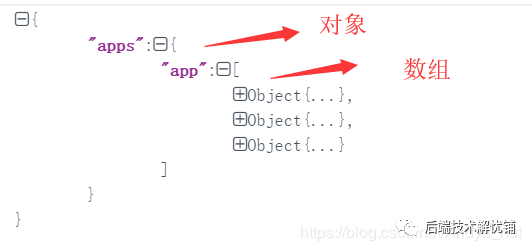
JSON转换对象
try {
//获取第一级对象
JSONObject appsJSONObject = JSON.parseObject(appsStr).getJSONObject("apps");
//判断是否为空
if (appsJSONObject == null || appsJSONObject .size() <= 0) {
log.info("json has no apps");
}
//获取第二级对象数组JSONArray
JSONArray appJSONArray = appsJSONObject .getJSONArray("app");
//转换成二级对象字符串
String appStr = JSON.toJSONString(appJSONArray );
//字符串转换成第二级对象数组List
List appList = new ArrayList<>();
appList = JSONObject.parseArray(appStr, Map.class);
log.info("length: {}", appList.size());
} catch (Exception e) {
log.error(e.getMessage());
}
常用三类转换
转换bean对象
//其他方式获取到的Object对象
Object obj = xxx;
String responseStr = JSON.toJSONString(obj);
XXXXBean xxxxBean = JSON.parseObject(responseStr, XXXXBean.class);
转换Map
Object obj = xxx;
String responseStr = JSON.toJSONString(obj);
Map map = JSONObject.parseObject(responseStr,Map.class);
转换List
Object obj = xxx;
String responseStr = JSON.toJSONString(obj);
List mapList = JSON.parseArray(responseStr , Map.class);
List xxxList = JSONObject.parseArray(responseStr, XXXBean.class);
FastJson API
序列化API
package com.alibaba.fastjson;
public abstract class JSON {
// 1、toJSONString():将Java对象object序列化为JSON字符串,支持各种各种Java基本类型和JavaBean
public static String toJSONString(Object object, SerializerFeature... features);
// 2、toJSONBytes():将Java对象object序列化为JSON字符串,按UTF-8编码返回JSON字符串bytes
public static byte[] toJSONBytes(Object object, SerializerFeature... features);
// 3、writeJSONString():将Java对象object序列化为JSON字符串,写入到Writer中
public static void writeJSONString(Writer writer,
Object object,
SerializerFeature... features);
// 4、writeJSONString():将Java对象object序列化为JSON字符串,按UTF-8编码写入到OutputStream中
public static final int writeJSONString(OutputStream os, //
Object object, //
SerializerFeature... features);
}
反序列化API
package com.alibaba.fastjson;
public abstract class JSON {
// 1、将JSON字符串jsonStr反序列化为JavaBean对象
public static T parseObject(String jsonStr,
Class clazz,
Feature... features);// 2、将JSON字节反序列化为JavaBean对象public static T parseObject(byte[] jsonBytes, // UTF-8格式的JSON字符串
Class clazz,
Feature... features);// 3、将JSON字符串反序列化为泛型类型的JavaBean对象public static T parseObject(String text,
TypeReference type,
Feature... features);// 4、将JSON字符串反序列为JSONObjectpublic static JSONObject parseObject(String text);
}
模板
序列化模板
JavaBean —> JSON String
import com.alibaba.fastjson.JSON;
XXXBean xxxBean = ...;
String jsonStr = JSON.toJSONString(xxxBean);
JavaBean —> JSON Bytes
import com.alibaba.fastjson.JSON;
XXXBean xxxBean = ...;
byte[] jsonBytes = JSON.toJSONBytes(xxxBean);
JavaBean —> JSON Writer
import com.alibaba.fastjson.JSON;
XXXBean xxxBean = ...;
Writer writer = ...;
JSON.writeJSONString(writer, xxxBean);
JavaBean —> JSON OutputStream
import com.alibaba.fastjson.JSON;
XXXBean xxxBean = ...;
OutputStream outputStream = ...;
JSON.writeJSONString(outputStream, xxxBean);
反序列化模板
JSON String —> JavaBean
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
String jsonStr = ...;
XXXBean xxxBean = JSON.parseObject(jsonStr, XXXBean.class);
JSON Bytes —> JavaBean
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
byte[] jsonBytes = ...;
XXXBean xxxBean = JSON.parseObject(jsonBytes, XXXBean.class);
JSON Generic Type —> JavaBean
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
String jsonStr = ...;
XXXBean xxxBean = JSON.parseObject(jsonStr, new TypeReference() {});
Type type = new TypeReference>() {}.getType();
List list = JSON.parseObject(jsonStr, type);
JSON String —> JavaBean
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
String jsonStr = ...;
JSONObject jsonObj = JSON.parseObject(jsonStr);